Assignment 2: Image Processing And Steganography
Part 1: Drawing Ellipses (16 Points)
Chris Tralie
Due Friday 9/23
In the first part of this assignment, we will draw a simple shape on a 2D grayscale image as a warmup to dynamic memory with 2D arrays. A grayscale image is a 2D array of type unsigned char
which has values between 0 and 255 at each entry, where 0 is black, 255 is white, and everything in between is shades of gray (for 256 possible values total). A shortcut for unsigned char
that you can use in this assignment is uint8_t
.
In this task, you'll be three different methods. Each method is specified in the file ellipse.h
provided in the starter code. Study the method specification provided in the method docstrings. You will then implement these methods in the associated ellipse.cpp
file as described below.
Also, here is a video on how to get started and how to manage tester files and object files:
Task 1 (4 Points)
uint8_t** allocateImage(int width, int height)
Create method to dynamically allocate a 2D array of the appropriate size for a grayscale image with height height
and width width
. Review the notes here on how to dynamically allocate 2D arrays.
Task 2 (4 Points)
void freeImage(uint8_t** image, int height)
Create a method to free all of the memory you allocated in task 1. Before you proceed, you should call allocateImage
and then call freeImage
in a small test file just to make sure you don't have a memory leak
Task 3 (4 Points)
void drawEllipse(uint8_t** image, int width, int height, float cx, float cy, float sx, float sy, uint8_t shade)
Draw an ellipse in a 2D array. Every pixel inside of the ellipse should be drawn with the grayscale value shade
, and those outside should be drawn with a color of 0. A pixel at location (x, y)
is on the inside of an ellipse centered at coordinates (cx, cy)
with axes of lengths sx
and sy
if
\[ \frac{(x - cx)^2}{sx^2} + \frac{(y - cy)^2}{sy^2} \leq 1 \]
Once you have these three methods done, you can make the program art
, and if you run it you should see the following image called art.png
in your assignment folder
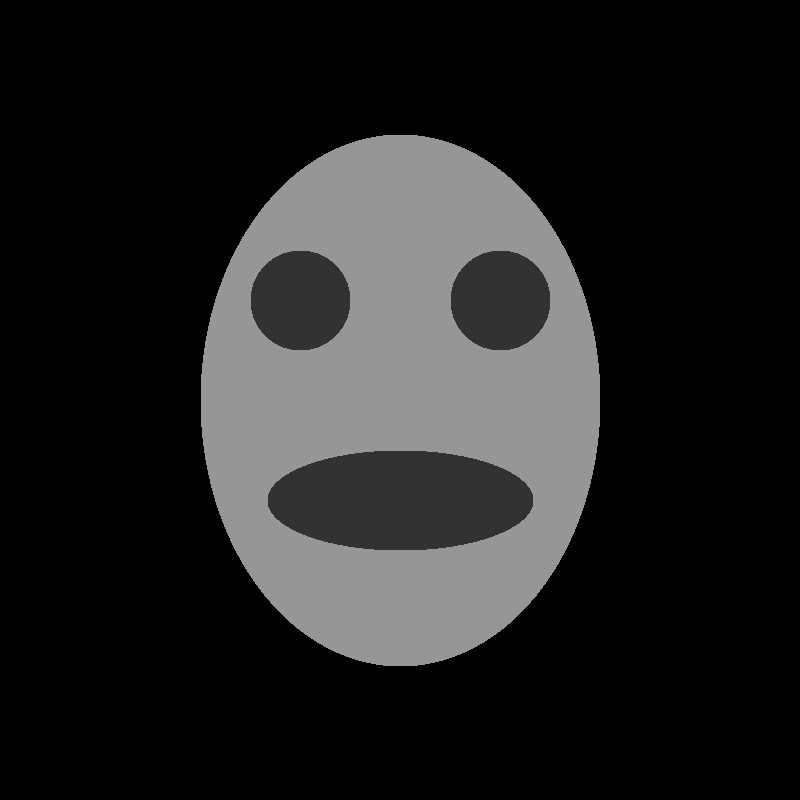
Task 4 (4 Points)
For the final task in this part of the assignment, create a program called drawEllipse
that includes ellipse.h
and links in the object file ellipse.o
, and which takes in 8 command line arguments:
-
filename
: A path to which to save the file. You can use the providedwriteImage
method, as shown inart.cpp
-
width
: The width of the image -
height
: The width of the image -
cx
: The x coordinate center in of the ellipse -
cy
: The y coordinate center in of the ellipse -
sx
: The x scale of the ellipse -
sy
: The y scale of the ellipse -
shade
: The shade of the ellipse
For example, running the program
should output the image ellipse1.png
that looks like this:
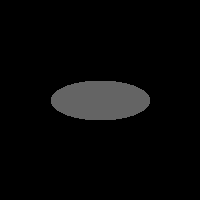
and running
should output the image ellipse2.png
that looks like this:
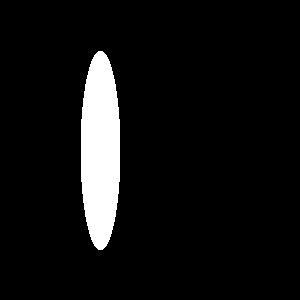
You may want to review the notes here on command line arguments. You can use atoi
to convert an argument to an int and atof
to convert a command line argument to a float.